Hi Friends,
Here I like to post the details of BPMN designer ( Business process management notation). Here I posted the jgraph code to achieve the BPMN Event types
File details:
- GraphViewer.java
- EventsFactory.java
- StartEvent.java
- EndEvent.java
- InterEvent.java
- EventsType.java
- EventDetails.java
- BPMNType.java
- BPMNEvent.java
1. GraphViewer.java
public class GraphViewer extends JFrame implements ActionListener
{
private JButton btnInsert = null;
private JButton btnDelete = null;
private JComboBox cbxEvent = null;
private JComboBox cbxObject = null;
public JGraph jGraph = null;
public DefaultGraphModel jGraphModel = null;
private static int id =0;
public GraphViewer()
{
super(“JGraph Demo-Kalaimaan”);
initGraph();
}
/**
*
*/
private void initGraph()
{
setLayout(new BorderLayout());
add(initNorthPanel(), BorderLayout.NORTH);
JScrollPane panel = new JScrollPane(initGraphPanel(),
JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED,
JScrollPane.HORIZONTAL_SCROLLBAR_AS_NEEDED);
add(panel, BorderLayout.CENTER);
setVisible(true);
setSize(new Dimension(600, 600));
}
/**
*
* @return
*/
private JComponent initNorthPanel()
{
JPanel temp = new JPanel(new BorderLayout());
JPanel child = new JPanel();
cbxObject = new JComboBox();
cbxObject.setPreferredSize(new Dimension(100, 25));
child.add(cbxObject);
cbxObject.addItem(BPMNType.START);
cbxObject.addItem(BPMNType.INTER);
cbxObject.addItem(BPMNType.END);
JLabel lab = new JLabel(” With “);
child.add(lab);
cbxEvent = new JComboBox();
cbxEvent.setPreferredSize(new Dimension(150, 25));
child.add(cbxEvent);
cbxEvent.addItem(BPMNEvent.MessageIcon);
cbxEvent.addItem(BPMNEvent.CancelIcon);
cbxEvent.addItem(BPMNEvent.CompensationIcon);
cbxEvent.addItem(BPMNEvent.ErrorIcon);
cbxEvent.addItem(BPMNEvent.LinkIcon);
cbxEvent.addItem(BPMNEvent.MultipleIcon);
cbxEvent.addItem(BPMNEvent.RuleIcon);
cbxEvent.addItem(BPMNEvent.TerminateIcon);
cbxEvent.addItem(BPMNEvent.TimerIcon);
cbxEvent.addItem(BPMNEvent.None);
btnInsert = new JButton(“Insert”);
btnInsert.addActionListener(this);
btnInsert.setPreferredSize(new Dimension(100, 25));
child.add(btnInsert);
btnDelete = new JButton(“Delete”);
btnDelete.addActionListener(this);
btnDelete.setPreferredSize(new Dimension(100, 25));
child.add(btnDelete);
temp.add(child);
return temp;
}
private JComponent initGraphPanel()
{
jGraphModel = new DefaultGraphModel();
jGraph = new JGraph(jGraphModel);
jGraph.setGridColor(Color.lightGray);
jGraph.setGridMode(JGraph.LINE_GRID_MODE);
jGraph.setGridSize(20);
jGraph.setGridEnabled(true);
jGraph.setGridVisible(true);
jGraph.setHandleColor(Color.red);
jGraph.setSelectionEnabled(true);
jGraph.getGraphLayoutCache().setFactory(new EventsFactory());
return jGraph;
}
@Override
public void actionPerformed(ActionEvent e)
{
if (e.getActionCommand().equalsIgnoreCase(“Insert”))
insertEvents();
else if (e.getActionCommand().equalsIgnoreCase(“Delete”))
deleteEvents();
}
private void insertEvents()
{
BPMNType type = (BPMNType) cbxObject.getSelectedItem();
BPMNEvent eve = (BPMNEvent) cbxEvent.getSelectedItem();
EventDetails dt = null;
switch (type)
{
case START:
dt = new EventDetails(type,”Start”, getId(), eve);
break;
case INTER:
dt = new EventDetails(type,”Inter”, getId(), eve);
break;
case END:
dt = new EventDetails(type,”Inter”, getId(), eve);
break;
}
DefaultGraphCell cell = new DefaultGraphCell(dt);
cell.setAttributes(getEventsAttributes());
jGraph.getGraphLayoutCache().insert(cell);
}
private void deleteEvents()
{
jGraph.getGraphLayoutCache().remove(jGraph.getSelectionCells());
jGraph.clearOffscreen();
}
private AttributeMap getEventsAttributes()
{
AttributeMap map = new AttributeMap();
Rectangle2D cellRect = new Rectangle(20, 20, 60, 60);
GraphConstants.setBounds(map, cellRect);
GraphConstants.setConstrained(map, true);
GraphConstants.setSizeable(map, false);
GraphConstants.setVerticalAlignment(map, JLabel.BOTTOM);
GraphConstants.setVerticalTextPosition(map, JLabel.CENTER);
GraphConstants.setGradientColor(map, Color.yellow.brighter());
GraphConstants.setBorderColor(map, Color.black);
GraphConstants.setBackground(map, Color.white);
GraphConstants.setOpaque(map, true);
GraphConstants.setEditable(map, false);
GraphConstants.setSelectable(map, true);
return map;
}
private String getId()
{
return “” + id++;
}
public static void main(String[] args)
{
try
{
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
}
catch (Exception e)
{
e.printStackTrace();
}
GraphViewer viewer = new GraphViewer();
viewer.setDefaultCloseOperation(DISPOSE_ON_CLOSE);
viewer.setVisible(true);
}
}
2. EventsFactory.java
public class EventsFactory extends DefaultCellViewFactory
{
public CellView createView(GraphModel model, Object cell)
{
CellView view = createVertexView(cell);
return view;
}
/**
*
*/
protected VertexView createVertexView(Object cell)
{
VertexView view = new VertexView(cell);
DefaultGraphCell graphCell = (DefaultGraphCell) cell;
EventDetails element = (EventDetails) graphCell.getUserObject();
if (element == null)
return view;
switch (element.getType())
{
case START:
view = new StartEvent(cell);
break;
case INTER:
view = new InterEvent(cell);
break;
case END:
view = new EndEvent(cell);
break;
}
return view;
}
}
3. StartEvent.java
public class StartEvent extends VertexView
{
public static EventsType eventTypes = new EventsType();
public static transient StartEventRenderer renderer = new StartEventRenderer();
public StartEvent()
{
super();
}
public StartEvent(Object cell)
{
super(cell);
}
public CellViewRenderer getRenderer()
{
return renderer;
}
public static class StartEventRenderer extends VertexRenderer
{
public void paint(Graphics g)
{
Graphics2D g2 = (Graphics2D) g;
Rectangle ovalRect = new Rectangle(1, 1, 39, 39);
if (super.isOpaque())
{
g2.setColor(super.getBackground());
if (gradientColor != null && !preview)
{
setOpaque(false);
g2.setPaint(new GradientPaint(0, 0, getBackground(), getWidth(), getHeight(),
gradientColor, true));
}
g2.fillOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
}
setBorder(null);
setOpaque(false);
Stroke old = g2.getStroke();
if (bordercolor != null)
{
g2.setColor(bordercolor);
g2.setStroke(new BasicStroke(1.7f));
g2.drawOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
g2.setStroke(old);
// set the event types..
EventDetails det = (EventDetails)((DefaultGraphCell)view.getCell()).getUserObject();
switch (det.getEvent())
{
case MessageIcon:
eventTypes.paintMessageIcon(g);
break;
case TimerIcon:
eventTypes.paintTimerIcon(g);
break;
case ErrorIcon:
eventTypes.paintErrorIcon(g);
break;
case CancelIcon:
eventTypes.paintCancelIcon(g);
break;
case CompensationIcon:
eventTypes.paintCompensationIcon(g);
break;
case RuleIcon:
eventTypes.paintRuleIcon(g);
break;
case LinkIcon:
eventTypes.paintLinkIcon(g);
break;
case TerminateIcon:
eventTypes.paintTerminateIcon(g);
break;
case MultipleIcon:
eventTypes.paintMultipleIcon(g);
break;
}
}
if (selected)
{
g2.setStroke(GraphConstants.SELECTION_STROKE);
g2.setColor(defaultBackground);
g2.setStroke(new BasicStroke(1.9f, BasicStroke.CAP_BUTT, BasicStroke.JOIN_MITER,
10.0f, new float[] { 5f, 5f }, 0.0f));
g2.drawOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
}
super.paint(g2);
}
}
}
4. EndEvent.java
public class EndEvent extends VertexView
{
public static EventsType eventTypes = new EventsType();
public static transient EndEventRenderer renderer = new EndEventRenderer();
public EndEvent()
{
super();
}
public EndEvent(Object cell)
{
super(cell);
}
public CellViewRenderer getRenderer()
{
return renderer;
}
public static class EndEventRenderer extends VertexRenderer
{
public void paint(Graphics g)
{
Graphics2D g2 = (Graphics2D) g;
Rectangle ovalRect = new Rectangle(2, 2, 36, 36);
if (super.isOpaque())
{
g2.setColor(super.getBackground());
if (gradientColor != null && !preview)
{
setOpaque(false);
g2.setPaint(new GradientPaint(0, 0, getBackground(), getWidth(), getHeight(),
gradientColor, true));
}
g2.fillOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
}
setBorder(null);
setOpaque(false);
Stroke old = g2.getStroke();
if (bordercolor != null)
{
g2.setColor(bordercolor);
g2.setStroke(new BasicStroke(4f));
g2.drawOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
g2.setStroke(old);
EventDetails det = (EventDetails)((DefaultGraphCell)view.getCell()).getUserObject();
switch (det.getEvent())
{
case MessageIcon:
eventTypes.paintMessageIcon(g);
break;
case TimerIcon:
eventTypes.paintTimerIcon(g);
break;
case ErrorIcon:
eventTypes.paintErrorIcon(g);
break;
case CancelIcon:
eventTypes.paintCancelIcon(g);
break;
case CompensationIcon:
eventTypes.paintCompensationIcon(g);
break;
case RuleIcon:
eventTypes.paintRuleIcon(g);
break;
case LinkIcon:
eventTypes.paintLinkIcon(g);
break;
case TerminateIcon:
eventTypes.paintTerminateIcon(g);
break;
case MultipleIcon:
eventTypes.paintMultipleIcon(g);
break;
}
}
if (selected)
{
g2.setStroke(GraphConstants.SELECTION_STROKE);
g2.setColor(defaultBackground);
g2.setStroke(new BasicStroke(4.5f, BasicStroke.CAP_BUTT, BasicStroke.JOIN_MITER,
10.0f, new float[] { 5f, 5f }, 0.0f));
g2.drawOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
g2.setStroke(old);
}
super.paint(g2);
}
}
}
5. InterEvent.java
public class InterEvent extends VertexView
{
public static EventsType eventTypes = new EventsType();
public static transient InterEventRenderer renderer = new InterEventRenderer();
public InterEvent()
{
super();
}
public InterEvent(Object cell)
{
super(cell);
}
public CellViewRenderer getRenderer()
{
return renderer;
}
public static class InterEventRenderer extends VertexRenderer
{
public void paint(Graphics g)
{
Graphics2D g2 = (Graphics2D) g;
Rectangle ovalRect = new Rectangle(1, 1, 39, 39);
if (super.isOpaque())
{
g2.setColor(super.getBackground());
if (gradientColor != null && !preview)
{
setOpaque(false);
g2.setPaint(new GradientPaint(0, 0, getBackground(), getWidth(), getHeight(),
gradientColor, true));
}
g2.fillOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
}
setBorder(null);
setOpaque(false);
Stroke old = g2.getStroke();
if (bordercolor != null)
{
g2.setColor(bordercolor);
g2.setStroke(new BasicStroke(1.7f));
g2.drawOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
g2.drawOval(ovalRect.x + 5, ovalRect.y + 5, ovalRect.width – 10,
ovalRect.height – 10);
g2.setStroke(old);
EventDetails det = (EventDetails)((DefaultGraphCell)view.getCell()).getUserObject();
switch (det.getEvent())
{
case MessageIcon:
eventTypes.paintMessageIcon(g);
break;
case TimerIcon:
eventTypes.paintTimerIcon(g);
break;
case ErrorIcon:
eventTypes.paintErrorIcon(g);
break;
case CancelIcon:
eventTypes.paintCancelIcon(g);
break;
case CompensationIcon:
eventTypes.paintCompensationIcon(g);
break;
case RuleIcon:
eventTypes.paintRuleIcon(g);
break;
case LinkIcon:
eventTypes.paintLinkIcon(g);
break;
case TerminateIcon:
eventTypes.paintTerminateIcon(g);
break;
case MultipleIcon:
eventTypes.paintMultipleIcon(g);
break;
}
}
if (selected)
{
g2.setStroke(GraphConstants.SELECTION_STROKE);
g2.setColor(defaultBackground);
g2.setStroke(new BasicStroke(1.9f, BasicStroke.CAP_BUTT, BasicStroke.JOIN_MITER,
10.0f, new float[] { 5f, 5f }, 0.0f));
g2.drawOval(ovalRect.x, ovalRect.y, ovalRect.width, ovalRect.height);
g2.setStroke(old);
}
super.paint(g2);
}
}
}
6. EventsType.java
public class EventsType
{
private Rectangle ovalRect = null;
private int hRadius = 18;
private int vRadius = 18;
public EventsType()
{
ovalRect = new Rectangle(0, 0, 40, 40);
}
/**
* Paint the Message Icon for the Event.
*/
public void paintMessageIcon(Graphics g)
{
if (g == null)
return;
Graphics2D g2 = (Graphics2D) g;
int x = ovalRect.x + (hRadius / 2) + 3;
int y = ovalRect.y + (vRadius / 2 + vRadius / 8) + 2;
g2.drawRect(x, y, hRadius – 1, vRadius * 3 / 4);
int xPoints[] = { x, x + hRadius – 1, x + ((hRadius – 1) / 2), x };
int yPoints[] = { y, y, y + (vRadius / 2), y };
g2.drawPolygon(xPoints, yPoints, 4);
}
/**
* Paint the Multiple Icon for the Event.
*/
public void paintMultipleIcon(Graphics g)
{
if (g == null)
return;
int side = (ovalRect.width – 8) * 4 / 10;
int x = ovalRect.x + ovalRect.width / 2;
int y = ovalRect.y + ovalRect.height / 2;
int triangle1x[] = { x – side, x, x + side };
int triangle1y[] = { y + side / 2, y – side, y + side / 2 };
int triangle2x[] = { x – side, x + side, x };
int triangle2y[] = { y – side / 2, y – side / 2, y + side };
g.fillPolygon(triangle1x, triangle1y, 3);
g.fillPolygon(triangle2x, triangle2y, 3);
}
/**
* Paint the Link Icon for the Event.
*/
public void paintLinkIcon(Graphics g)
{
if (g == null)
return;
int x = ovalRect.x + 7;
int y = ovalRect.y + 7;
int width = ovalRect.width – 12;
int height = ovalRect.height – 14;
int xPoints[] = { x, x + width * 6 / 10, x + width * 6 / 10, x + width, x + width * 6 / 10,
x + width * 6 / 10, x, x };
int yPoints[] = { y + height / 4, y + height / 4, y, y + height / 2, y + height,
y + height * 3 / 4, y + height * 3 / 4, y + height / 4 };
g.fillPolygon(xPoints, yPoints, 8);
}
/**
* Paint the Rule Icon for the Event.
*/
public void paintRuleIcon(Graphics g)
{
if (g == null)
return;
Graphics2D g2 = (Graphics2D) g;
int x = ovalRect.x + (hRadius / 2) + 4;
int y = ovalRect.y + (hRadius / 2) + 3;
g2.drawRect(x, y, hRadius – 3, vRadius – 2);
int lineWidth = hRadius – 7;
int spaceBetweenLines = (vRadius – 3) / 4;
int lineX = x + 2;
int lineY = y + spaceBetweenLines – 1;
for (int i = 0; i < 4; i++)
{
g.drawLine(lineX, lineY, lineX + lineWidth, lineY);
lineY = lineY + spaceBetweenLines;
}
}
/**
* Paint the Timer Icon for the Event.
*/
public void paintTimerIcon(Graphics g)
{
if (g == null)
return;
final float radPerSecMin = (float) (Math.PI / 30.0);
final float threePi = (float) (3.0 * Math.PI);
Graphics2D g2 = (Graphics2D) g;
g2.setColor(Color.black);
int x = ovalRect.x + 8;
int y = ovalRect.y + 8;
int width = ovalRect.width – 16;
int height = ovalRect.height – 16;
// circle
g2.drawOval(x, y, width, height);
int secondRadius = width / 2;
int minuteRadius = secondRadius * 3 / 4;
int hourRadius = secondRadius / 2;
// minute hand
float minuteAngle = threePi – (radPerSecMin * 4.0f);
drawRadius(g, x + width / 2, y + height / 2, minuteAngle, 0, minuteRadius);
// hour hand
float hourAngle = threePi – (5 * radPerSecMin * 3.0f);
drawRadius(g, x + width / 2, y + height / 2, hourAngle, 0, hourRadius);
// To indicate the hours around the circle
int ticStart;
for (int sec = 0; sec < 60; sec += 5)
{
ticStart = width * 4 / 10;
drawRadius(g, x + width / 2, y + height / 2, radPerSecMin * sec, ticStart, width / 2);
}
}
/**
* Paint the Hour indicator around the Clock for the Event.
*/
private void drawRadius(Graphics g, int x, int y, double angle, int minRadius, int maxRadius)
{
if (g == null)
return;
float sine = (float) Math.sin(angle);
float cosine = (float) Math.cos(angle);
int dxmin = (int) (minRadius * sine);
int dymin = (int) (minRadius * cosine);
int dxmax = (int) (maxRadius * sine);
int dymax = (int) (maxRadius * cosine);
g.drawLine(x + dxmin, y + dymin, x + dxmax, y + dymax);
}
/**
* Paint the Error Icon for the Event.
*/
public void paintErrorIcon(Graphics g)
{
if (g == null)
return;
int x = ovalRect.x + 10;
int y = ovalRect.y + 10;
int width = ovalRect.width – 18;
int height = ovalRect.height – 18;
int triangle1x[] = { x, x + width / 6, x + width / 3 };
int triangle1y[] = { y + height, y + height / 6, y + height / 3 };
int triangle2x[] = { x + width, x + 5 * width / 6, x + 2 * width / 3 };
int triangle2y[] = { y, y + 5 * height / 6, y + 2 * height / 3 };
int polyx[] = { x + width / 3, x + (width / 3) – (width / 10), x + 2 * width / 3,
x + (2 * width / 3) + (width / 10) };
int polyy[] = { y + height / 3, y + (height / 3) + (height / 10), y + 2 * height / 3,
y + (2 * height / 3) – (height / 10) };
g.fillPolygon(triangle1x, triangle1y, 3);
g.fillPolygon(triangle2x, triangle2y, 3);
g.fillPolygon(polyx, polyy, 4);
}
/**
* Paint the Cancel Icon for the Event.
*/
public void paintCancelIcon(Graphics g)
{
if (g == null)
return;
int x = ovalRect.x + 8;
int y = ovalRect.y + 8;
int side = ovalRect.width – 16;
int n = side * 2 / 10;
int rect1x[] = { x, x + n, x + side, x + side – n };
int rect1y[] = { y + n, y, y + side – n, y + side };
int rect2x[] = { x, x + side – n, x + side, x + n };
int rect2y[] = { y + side – n, y, y + n, y + side };
g.fillPolygon(rect1x, rect1y, 4);
g.fillPolygon(rect2x, rect2y, 4);
}
/**
* Paint the Compensation Icon for the Event.
*/
public void paintCompensationIcon(Graphics g)
{
if (g == null)
return;
int x = ovalRect.x + 8;
int y = ovalRect.y + ovalRect.height / 2;
int side = ovalRect.width / 2;
int tx1Points[] = { x, x + side / 2, x + side / 2, x };
int ty1points[] = { y, y – side / 2, y + side / 2, y };
x = x + side / 2;
int tx2Points[] = { x, x + side / 2, x + side / 2, x };
int ty2points[] = { y, y – side / 2, y + side / 2, y };
g.fillPolygon(tx1Points, ty1points, 4);
g.fillPolygon(tx2Points, ty2points, 4);
}
/**
* Paint the Terminate Icon for the Event.
*/
public void paintTerminateIcon(Graphics g)
{
if (g == null)
return;
g.fillOval(ovalRect.x + 8, ovalRect.y + 8, ovalRect.width – 16, ovalRect.height – 16);
}
}
7. EventDetails.java
public class EventDetails
{
private BPMNType cellType;
private BPMNEvent cellEvent;
private String label = “”;
private String id = “”;
public EventDetails(BPMNType type, String lab, String idE, BPMNEvent event)
{
cellEvent = event;
cellType = type;
label = lab;
id = idE;
}
public BPMNType getType()
{
return cellType;
}
public BPMNEvent getEvent()
{
return cellEvent;
}
public String getLabel()
{
return label;
}
public String getId()
{
return id;
}
}
8. BPMNType.java
public enum BPMNType
{
START,END,INTER
}
9. BPMNEvent.java
public enum BPMNEvent
{
MessageIcon, MultipleIcon, LinkIcon, RuleIcon, TimerIcon,
ErrorIcon, CancelIcon, CompensationIcon, TerminateIcon, None
}
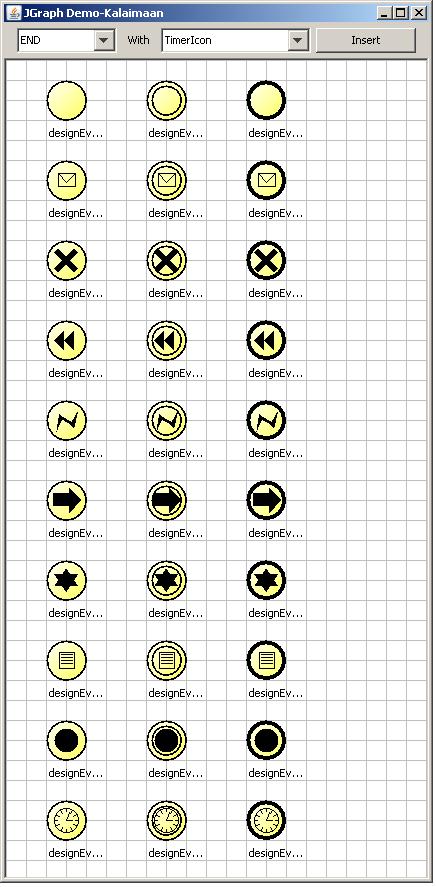